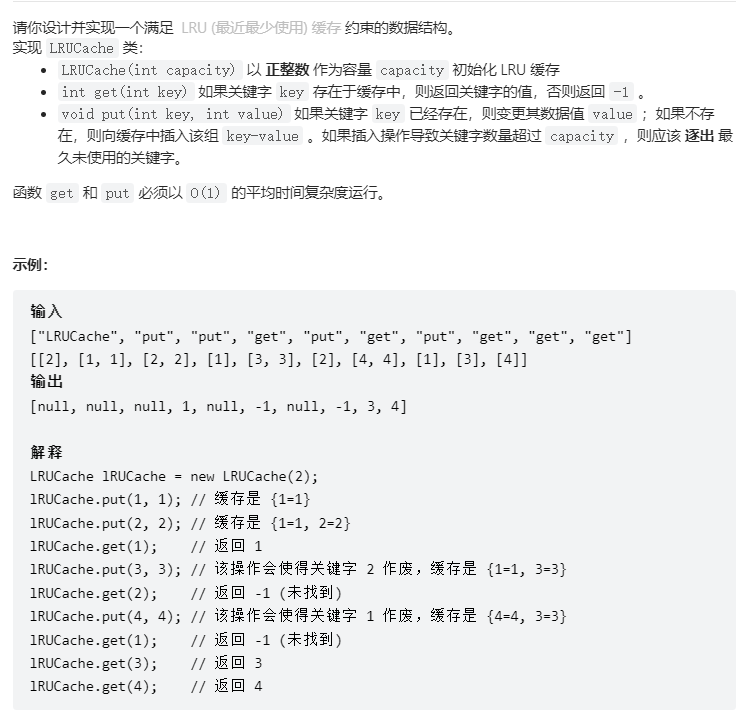
class LRUCache {
private:
unordered_map <int ,list<pair<int,int>>::iterator>mp;
list<pair<int,int>> cache;
int capacity;
public:
LRUCache(int capacity) {
this->capacity=capacity;
}
int get(int key) {
if(mp.find(key)==mp.end())return -1;
else {
auto key_value=*mp[key];
cache.erase(mp[key]);
cache.push_front(key_value);
mp[key]=cache.begin();
return key_value.second;
}
}
void put(int key, int value) {
if(mp.find(key)==mp.end()){
if(cache.size()==capacity){
mp.erase(cache.back().first);
cache.pop_back();
}
}else{
cache.erase(mp[key]);
}
cache.push_front({key,value});
mp[key]=cache.begin();
}
};